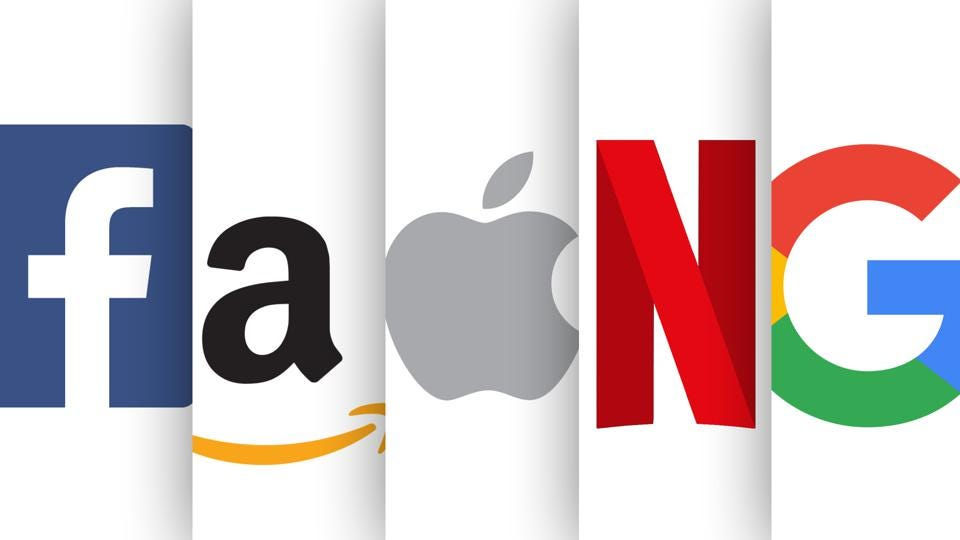
1. Introduction
Machine learning (ML) has quickly become one of the most in-demand fields in the tech industry, with companies like Google, Amazon, and Meta constantly seeking talented engineers to drive innovation. As a result, ML interviews at these top-tier companies are highly competitive and rigorous. Candidates need to demonstrate not only technical skills but also the ability to approach complex problems with creativity and efficiency.
Preparing for these interviews requires a holistic approach. Companies often test candidates in multiple areas, including coding, system design, ML theory, and behavioral questions to assess cultural fit. This blog serves as a comprehensive guide to the 50 most frequently asked ML interview questions that cover all these categories. With detailed answers and explanations, we aim to help you get ready for your next big ML interview and maximize your chances of success.
2. Why Preparation is Key for ML Interviews at Top Companies
Securing a job in machine learning at a leading tech company isn’t just about having advanced degrees or understanding ML algorithms—it’s about how you perform under pressure, how well you communicate complex ideas, and how you solve real-world problems using the right technical tools. Companies like Google, Amazon, and Apple are known for their thorough and structured interview processes, where a single mistake can mean losing the opportunity.
In addition to technical proficiency, these companies value engineers who can design scalable, efficient systems and collaborate effectively with cross-functional teams. This is why ML interviews are often divided into several categories: coding challenges, system design problems, ML domain-specific questions, and behavioral questions. Each aspect of the interview evaluates a different skill set, and being unprepared in any area can diminish your overall performance.
Moreover, top companies focus on hiring candidates who are not only technically sound but also fit well within the company’s culture. They look for individuals who can thrive in collaborative environments, handle ambiguity, and display leadership potential. By thoroughly preparing for all the different question types, you’ll increase your chances of performing well in the interview and standing out from other candidates.
In the following sections, we’ll dive into each category and go over 50 key questions commonly asked during ML interviews at top-tier companies, providing detailed answers and guidance on how to approach them.
3. Coding and Algorithms Questions
In machine learning interviews, top companies expect candidates to demonstrate a strong foundation in coding and algorithmic thinking. You'll often be asked to solve algorithmic problems on the spot, write efficient code, and explain your approach. Below are 15 common coding questions that have appeared in ML interviews at top-tier companies, along with detailed answers and explanations.
1. Implement Logistic Regression from scratch.
Problem: Write a Python function to implement logistic regression using gradient descent.
Solution: Logistic regression is a classification algorithm that maps input features to a probability value using the sigmoid function. The key steps involve:
Initializing weights and biases.
Using the sigmoid function to calculate predictions.
Calculating the loss using binary cross-entropy.
Updating weights using gradient descent.
import numpy as np
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def logistic_regression(X, y, lr=0.01, epochs=1000):
m, n = X.shape
weights = np.zeros(n)
bias = 0
for _ in range(epochs):
z = np.dot(X, weights) + bias
predictions = sigmoid(z)
# Compute gradients
dw = (1/m) * np.dot(X.T, (predictions - y))
db = (1/m) * np.sum(predictions - y)
# Update weights and bias
weights -= lr * dw
bias -= lr * db
return weights, bias
Explanation:
We initialize weights and biases to zero.
The sigmoid function is used to transform the linear combination of inputs into a probability.
Gradient descent is used to update the weights based on the gradient of the loss function.
2. Find the top K frequent elements in a list using a heap.
Problem: Given a list of integers, return the K most frequent elements.
Solution: You can solve this using a max-heap. The idea is to count the frequency of each element and then maintain a heap of size K with the most frequent elements.
from collections import Counter
import heapq
def top_k_frequent(nums, k):
freq = Counter(nums)
return heapq.nlargest(k, freq.keys(), key=freq.get)
Explanation:
First, we count the frequency of each element using the Counter from the collections module.
Then, heapq.nlargest() is used to return the K most frequent elements based on their frequency.
3. Design a function to perform matrix multiplication.
Problem: Write a Python function to perform matrix multiplication between two matrices.
Solution: Matrix multiplication involves computing the dot product between rows of the first matrix and columns of the second matrix.
def matrix_multiplication(A, B):
result = [[0 for in range(len(B[0]))] for in range(len(A))]
for i in range(len(A)):
for j in range(len(B[0])):
for k in range(len(B)):
result[i][j] += A[i][k] * B[k][j]
return result
Explanation:
We initialize an empty result matrix.
Nested loops are used to calculate the dot product for each element in the result matrix.
4. Reverse a linked list.
Problem: Reverse a singly linked list.
Solution: This is a common coding problem, where you iterate through the linked list and reverse the pointers.
class ListNode:
def init(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
Explanation:
We iterate through the list, reversing the next pointers one node at a time, and return the new head of the list.
5. Find the longest common subsequence between two strings.
Problem: Given two strings, find the length of their longest common subsequence.
Solution: This can be solved using dynamic programming.
def longest_common_subsequence(s1, s2):
m, n = len(s1), len(s2)
dp = [[0] * (n+1) for _ in range(m+1)]
for i in range(1, m+1):
for j in range(1, n+1):
if s1[i-1] == s2[j-1]:
dp[i][j] = dp[i-1][j-1] + 1
else:
dp[i][j] = max(dp[i-1][j], dp[i][j-1])
return dp[m][n]
Explanation:
We use a 2D DP array where dp[i][j] represents the length of the longest common subsequence up to the i-th character of s1 and the j-th character of s2.
6. Check if a string is a valid palindrome.
Problem: Given a string, check if it reads the same forward and backward, ignoring spaces and punctuation.
Solution: We can use two pointers to compare characters from both ends of the string.
def is_palindrome(s):
s = ''.join(e for e in s if e.isalnum()).lower()
return s == s[::-1]
Explanation:
We first sanitize the input string by removing non-alphanumeric characters and converting it to lowercase.
Then, we check if the string is equal to its reverse.
7. Implement K-nearest neighbors algorithm.
Problem: Write a Python function to implement the K-nearest neighbors (KNN) algorithm.
Solution: KNN is a simple, non-parametric algorithm that classifies a point based on the majority class of its K nearest neighbors.
import numpy as np
from collections import Counter
def knn(X_train, y_train, X_test, k):
distances = np.sqrt(((X_train - X_test)**2).sum(axis=1))
nearest_indices = np.argsort(distances)[:k]
nearest_labels = y_train[nearest_indices]
return Counter(nearest_labels).most_common(1)[0][0]
Explanation:
We calculate the Euclidean distance between the test point and all training points.
The K nearest points are identified, and the majority label among them is returned as the prediction.
8. Merge two sorted linked lists.
Problem: Merge two sorted linked lists into a single sorted list.
Solution: We can iterate through both linked lists simultaneously and merge them.
def merge_two_sorted_lists(l1, l2):
dummy = ListNode()
current = dummy
while l1 and l2:
if l1.val < l2.val:
current.next = l1
l1 = l1.next
else:
current.next = l2
l2 = l2.next
current = current.next
current.next = l1 if l1 else l2
return dummy.next
Explanation:
We use a dummy node to simplify list merging and iterate through both lists, appending the smaller node to the result.
9. Find the first non-repeating character in a string.
Problem: Given a string, find the first character that does not repeat.
Solution: We can use a dictionary to store character counts and iterate over the string to find the first character with a count of 1.
from collections import Counter
def first_non_repeating_char(s):
freq = Counter(s)
for char in s:
if freq[char] == 1:
return char
return None
Explanation:
We use Counter to count the frequency of each character, then find the first character with a count of 1.
4. System Design Questions
In machine learning interviews at top-tier companies, system design questions often focus on building scalable ML systems, pipelines, or infrastructure that can handle vast amounts of data. These questions assess your ability to architect efficient and scalable systems while considering aspects like data flow, storage, computation, and communication between components. Below are 10 frequently asked system design questions in ML interviews, along with guidance on how to approach them.
1. Design a Recommendation System for an E-commerce Platform
Problem: You are tasked with designing a recommendation system for an e-commerce platform (like Amazon) that provides personalized product recommendations to users.
Approach:
Key Components:
Data Collection: Gather user data (browsing history, past purchases, clicks, ratings).
Feature Engineering: Create user profiles based on their behavior and extract product features (categories, price range, popularity).
Modeling: Use a hybrid recommendation approach:
Collaborative Filtering for user-to-user and item-to-item recommendations.
Content-based Filtering for suggesting similar products based on past preferences.
Infrastructure: Ensure scalability with a distributed architecture, using technologies like Apache Kafka for data streaming and Spark for batch processing.
Real-Time Recommendations: For real-time suggestions, use an approximate nearest neighbors algorithm like FAISS (Facebook AI Similarity Search).
Considerations: Handling cold-start users (no historical data), scaling to millions of users, model retraining frequency, and A/B testing for evaluating recommendation efficacy.
2. Build a Distributed Training System for Deep Learning Models
Problem: Design a system to distribute the training of a deep learning model (e.g., for image recognition) across multiple machines.
Approach:
Key Components:
Data Partitioning: Use techniques like data parallelism (splitting data across multiple GPUs/machines) or model parallelism (splitting the model itself).
Parameter Synchronization: Use parameter servers to coordinate the training process by synchronizing model parameters between workers.
Communication: Implement efficient communication protocols (e.g., gRPC or MPI) to minimize overhead and reduce training time.
Frameworks: Use distributed training frameworks like TensorFlow Distributed, PyTorch Distributed, or Horovod to manage the workload.
Considerations: Fault tolerance (how to handle machine failures), load balancing between workers, and ensuring that data transfer doesn’t become a bottleneck.
3. Design a Real-Time Fraud Detection System
Problem: Build a system that detects fraudulent transactions in real-time for a financial institution.
Approach:
Key Components:
Data Pipeline: Stream incoming transactions in real-time using a messaging queue (e.g., Apache Kafka or AWS Kinesis).
Feature Engineering: Engineer features like transaction history, geographic location, device type, and frequency of transactions.
Modeling: Use supervised learning models like Random Forests or XGBoost trained on historical transaction data, with labels indicating fraud vs. non-fraud.
Real-Time Inference: Deploy the model as a microservice using a lightweight, low-latency platform (e.g., Flask + Gunicorn).
Feedback Loop: Implement a feedback mechanism to continuously update the model with new fraud cases.
Considerations: Low latency requirements, false positives vs. false negatives, handling imbalanced datasets (fraud is rare), and regulatory constraints.
4. Design a Scalable Feature Store for Machine Learning Models
Problem: Design a system to store and manage machine learning features that can be reused across multiple models and teams.
Approach:
Key Components:
Data Ingestion: Collect features from batch sources (data warehouses) and real-time streams.
Feature Storage: Use a combination of online stores (low-latency databases like Redis or DynamoDB) for real-time serving and offline stores (like BigQuery or S3) for batch processing.
Feature Transformation: Create reusable transformations (e.g., scaling, encoding) that can be consistently applied across models.
Versioning: Maintain version control for features to ensure reproducibility during model retraining.
Considerations: Managing data consistency between online and offline stores, ensuring low-latency retrieval, and scaling the system to handle hundreds or thousands of features.
5. Build a Data Pipeline for Model Training and Deployment
Problem: You are asked to design a data pipeline that automates the process of collecting, cleaning, training, and deploying ML models.
Approach:
Key Components:
Data Ingestion: Use ETL processes to extract data from various sources (e.g., relational databases, APIs), clean it, and store it in a data lake or warehouse (e.g., AWS S3).
Feature Engineering: Automate feature extraction and transformation using a pipeline tool like Airflow or Luigi.
Model Training: Use containerized environments (Docker) to run model training jobs on cloud infrastructure (e.g., AWS SageMaker or Google AI Platform).
Model Deployment: Deploy models to a scalable inference environment (e.g., Kubernetes or serverless platforms).
Considerations: Scalability, automation of model versioning, A/B testing for new model deployments, and monitoring system performance.
6. Design a Search Engine for Large-Scale Document Retrieval
Problem: Build a search engine for retrieving documents from a large-scale dataset (e.g., millions of research papers or blog articles).
Approach:
Key Components:
Indexing: Use an inverted index to store mappings between words and their occurrences in documents. Tools like Elasticsearch or Apache Solr are commonly used for this purpose.
Ranking: Implement ranking algorithms based on TF-IDF (Term Frequency-Inverse Document Frequency) or use a learned ranking model for more complex queries.
Scaling: Use sharding and replication to scale the system horizontally.
Query Processing: Optimize query parsing to handle complex search queries (e.g., wildcards, fuzzy matching).
Considerations: Handling billions of documents, ensuring fast query response times, and updating the index in near real-time.
7. Build a Data Lake for Storing Unstructured Data
Problem: Design a scalable data lake to store unstructured data (e.g., text, images, audio) that can later be used for training ML models.
Approach:
Key Components:
Storage Layer: Use cloud-based storage solutions (e.g., AWS S3 or Google Cloud Storage) to store raw, unstructured data.
Metadata Management: Implement a metadata layer to track data schemas, timestamps, and source information.
Data Access: Provide access to the data lake using APIs or query engines like Presto or Athena.
Security: Ensure the system adheres to privacy and security standards (e.g., encryption, role-based access).
Considerations: Handling large-scale, diverse data formats, ensuring data quality and integrity, and scaling as data grows.
8. Design an Online Learning System for Real-Time Model Updates
Problem: Build a system that allows machine learning models to learn and update continuously in real-time with new incoming data.
Approach:
Key Components:
Data Stream: Use Kafka or another streaming platform to continuously feed data into the system.
Incremental Learning: Choose algorithms that support online learning, such as stochastic gradient descent (SGD) or Hoeffding trees for decision-making.
Model Update: Implement mechanisms for updating model weights incrementally without retraining from scratch.
Deployment: Use a microservice architecture for deploying real-time updated models.
Considerations: Handling concept drift, ensuring model stability with new data, and managing latency in model updates.
9. Design a Model Monitoring System to Track ML Model Performance
Problem: Design a system to continuously monitor machine learning models in production and detect any degradation in performance.
Approach:
Key Components:
Data Collection: Continuously collect real-time data on model inputs and outputs.
Performance Metrics: Track key metrics like accuracy, precision/recall, and latency.
Alerts: Set up alerts for anomalies, such as performance degradation or data drift, using monitoring tools (e.g., Prometheus, Grafana).
Feedback Loop: Implement automated retraining or rollback mechanisms when performance drops below a threshold.
Considerations: Real-time alerting, dealing with false positives in monitoring, and ensuring smooth model retraining and redeployment.
10. Design an ML Model Marketplace
Problem: Build a platform where users can upload, share, and access machine learning models, similar to TensorFlow Hub or Hugging Face Model Hub.
Approach:
Key Components:
Model Upload: Provide an API or interface for users to upload pre-trained models.
Model Search and Discovery: Implement a search engine that allows users to find models based on task, architecture, or dataset.
Version Control: Keep track of model versions and ensure reproducibility.
Model Deployment: Offer one-click deployment options for users who want to integrate the models into their own applications.
Considerations: Model security, licensing, ensuring that models meet performance and accuracy standards, and scaling the platform.
5. Machine Learning Domain Questions
In the ML domain section of the interview, top companies focus on evaluating your theoretical understanding of machine learning concepts, algorithms, and the ability to apply them to real-world problems. These questions assess your depth of knowledge in ML theory, algorithmic trade-offs, and practical implementation strategies. Below are 15 commonly asked ML domain questions, along with detailed explanations.
1. Explain the difference between L1 and L2 regularization.
Answer: L1 and L2 regularization are techniques used to prevent overfitting by adding a penalty to the loss function based on the weights of the model.
L1 Regularization (Lasso): Adds the absolute value of the weights as a penalty: λ∑∣w∣\lambda \sum |w|λ∑∣w∣. This tends to produce sparse weight vectors, meaning that many weights are zero. This is useful for feature selection because it effectively ignores less important features.
L2 Regularization (Ridge): Adds the square of the weights as a penalty: λ∑w2\lambda \sum w^2λ∑w2. L2 regularization doesn’t drive weights to zero but rather reduces their magnitude. It is less likely to completely ignore any feature but helps distribute the weights more evenly across features.
When to use:
Use L1 regularization when feature selection is desired, or you expect many irrelevant features.
Use L2 regularization when you don’t want sparsity but prefer to penalize large weights more heavily.
2. What is the curse of dimensionality? How does it affect ML models?
Answer: The "curse of dimensionality" refers to the various phenomena that arise when analyzing and organizing data in high-dimensional spaces (i.e., spaces with many features). As the number of dimensions increases, the volume of the space increases exponentially, making the data sparse.
Effects on ML models:
Increased computational cost: High-dimensional data requires more computation, memory, and storage.
Sparsity: In high-dimensional space, data points are further apart, making it difficult for machine learning models to identify patterns or clusters.
Overfitting: With many features, models may fit the noise in the data instead of the actual signal, leading to poor generalization on new data.
Solutions:
Dimensionality reduction techniques like Principal Component Analysis (PCA) or t-SNE.
Feature selection: Removing irrelevant or redundant features can reduce the dimensionality.
3. Describe the working of the Gradient Boosting algorithm.
Answer: Gradient Boosting is an ensemble learning method that builds models sequentially, where each new model corrects the errors made by the previous models. It is primarily used for both regression and classification tasks.
Steps:
Initialize the model with a simple base model (e.g., a single constant prediction).
Calculate residuals: At each step, compute the residual errors (the difference between the actual value and the prediction).
Fit a new model: Train a new model to predict the residuals. This new model focuses on reducing the errors from the previous one.
Update the prediction: Add the predictions from the new model to the previous model's predictions.
Repeat the process for a predefined number of iterations or until a stopping criterion is met.
Advantages: Gradient boosting often results in highly accurate models. Variants like XGBoost and LightGBM are known for their efficiency and performance in practical use cases.
Disadvantages: Gradient boosting can be prone to overfitting if not properly tuned, and it’s computationally expensive compared to simpler models.
4. What is a confusion matrix, and how is it used to evaluate a model?
Answer: A confusion matrix is a performance measurement tool for classification problems. It shows how many of the predictions made by a model were correct and incorrect, by comparing the predicted labels with the actual labels.
Structure:
True Positives (TP): Correctly predicted positive observations.
True Negatives (TN): Correctly predicted negative observations.
False Positives (FP): Incorrectly predicted as positive (Type I error).
False Negatives (FN): Incorrectly predicted as negative (Type II error).
Usage:
Accuracy: TP+TNTP+TN+FP+FN\frac{TP + TN}{TP + TN + FP + FN}TP+TN+FP+FNTP+TN (overall correct predictions).
Precision: TPTP+FP\frac{TP}{TP + FP}TP+FPTP (how many positive predictions were correct).
Recall: TPTP+FN\frac{TP}{TP + FN}TP+FNTP (how many actual positives were correctly predicted).
F1 Score: The harmonic mean of precision and recall, useful when dealing with imbalanced datasets.
5. What is overfitting and underfitting in ML? How can they be mitigated?
Answer:
Overfitting: Occurs when a model is too complex and fits the noise in the training data rather than the underlying pattern. This results in excellent performance on the training data but poor performance on new, unseen data.
Underfitting: Happens when the model is too simple and cannot capture the underlying pattern in the data, leading to poor performance on both training and test data.
Mitigation strategies:
For overfitting:
Regularization (L1/L2): Adds a penalty to the model for having large weights.
Cross-validation: Ensures the model generalizes well across different subsets of data.
Pruning: For decision trees, reducing the complexity by trimming branches that offer little gain.
Early stopping: Stops training the model when performance on the validation set starts to degrade.
For underfitting:
Increase model complexity: Use more complex models (e.g., deeper neural networks).
Add features: Introduce new features to capture more information from the data.
6. Explain the bias-variance tradeoff in machine learning.
Answer: The bias-variance tradeoff refers to the balance between two sources of error in machine learning models:
Bias: Error due to overly simplistic assumptions made by the model. High bias leads to underfitting.
Variance: Error due to the model’s sensitivity to small fluctuations in the training data. High variance leads to overfitting.
Tradeoff:
A model with high bias may miss relevant information (underfitting), while a model with high variance may learn irrelevant details (overfitting).
The goal is to find a balance where both bias and variance are minimized to ensure good performance on unseen data.
Solutions:
Regularization: Adds penalties for overly complex models to reduce variance.
Cross-validation: Helps in tuning models to achieve the right balance between bias and variance.
7. What is AUC-ROC, and how do you interpret it?
Answer: AUC-ROC (Area Under the Receiver Operating Characteristic Curve) is a performance measurement for classification problems at various threshold settings.
ROC Curve: Plots the True Positive Rate (Recall) against the False Positive Rate at different threshold levels.
AUC: The area under the ROC curve. It represents the probability that the classifier will rank a randomly chosen positive instance higher than a randomly chosen negative instance.
Interpretation:
AUC = 1: Perfect classifier.
AUC > 0.9: Excellent model.
AUC between 0.7 and 0.9: Good model.
AUC = 0.5: No better than random guessing.
8. What is cross-validation, and why is it important?
Answer: Cross-validation is a technique used to assess how a machine learning model will generalize to an independent dataset. It divides the data into several subsets (folds), trains the model on some folds, and tests it on the remaining fold. The process is repeated for different folds.
Types:
K-Fold Cross-Validation: The data is divided into K subsets, and the model is trained K times, each time leaving out one subset for testing.
Leave-One-Out Cross-Validation (LOOCV): Each data point is used once as the validation set while the rest are used for training.
Importance:
It helps detect overfitting by ensuring the model performs well across different data splits.
It provides a more reliable estimate of model performance compared to a single train-test split.
9. Explain the concept of precision and recall, and when would you prefer one over the other?
Answer:
Precision: Measures the accuracy of positive predictions. It’s the ratio of true positives to the sum of true and false positives: Precision=TPTP+FP\text{Precision} = \frac{TP}{TP + FP}Precision=TP+FPTP.
Recall (Sensitivity): Measures the ability of a model to find all the relevant cases. It’s the ratio of true positives to the sum of true positives and false negatives: Recall=TPTP+FN\text{Recall} = \frac{TP}{TP + FN}Recall=TP+FNTP.
When to prefer one over the other:
Use precision when the cost of false positives is high. For example, in spam detection, you want to minimize the number of legitimate emails marked as spam.
Use recall when the cost of false negatives is high. For example, in medical diagnosis, you want to minimize the number of actual diseases that go undetected.
10. What is transfer learning, and how is it used in machine learning?
Answer: Transfer learning is a technique where a model trained on one task is reused for a different but related task. This is commonly used in deep learning, especially in domains like image recognition or natural language processing.
How it works:
You take a pre-trained model (like ResNet or BERT) that has been trained on a large dataset (e.g., ImageNet for images or Wikipedia for text).
You then fine-tune the model on your specific task by retraining it on a smaller dataset, while leveraging the already learned features.
Advantages:
Reduces the amount of training data needed.
Shortens training time.
Often leads to better performance, especially when labeled data is scarce.
11. What is the difference between bagging and boosting?
Answer: Bagging and boosting are both ensemble learning techniques that combine multiple models to improve overall performance, but they have key differences in how they create and combine models.
Bagging (Bootstrap Aggregating):
Process: In bagging, multiple models (usually decision trees) are trained independently on different subsets of the training data (created through bootstrapping, i.e., random sampling with replacement). The final prediction is made by averaging (for regression) or voting (for classification) over all models.
Purpose: Bagging helps to reduce variance and prevent overfitting.
Example: Random Forest is a popular bagging algorithm.
Boosting:
Process: In boosting, models are trained sequentially, where each new model focuses on correcting the errors made by the previous models. The final prediction is made by a weighted combination of all models. Unlike bagging, boosting assigns higher weights to misclassified instances, so the next model pays more attention to those errors.
Purpose: Boosting reduces bias and helps improve weak learners.
Example: AdaBoost, Gradient Boosting, and XGBoost are popular boosting algorithms.
When to use:
Use bagging when the goal is to reduce variance (e.g., for high-variance models like decision trees).
Use boosting when the goal is to reduce bias and improve the model’s accuracy.
12. What is a convolutional neural network (CNN), and how is it used?
Answer: A Convolutional Neural Network (CNN) is a specialized type of deep neural network designed primarily for processing structured grid-like data, such as images. CNNs are widely used in computer vision tasks like image classification, object detection, and facial recognition.
Key Components:
Convolutional Layers: These layers apply filters (kernels) to input images to detect various features like edges, textures, or shapes. Each filter scans the image, creating a feature map.
Pooling Layers: Pooling layers reduce the spatial dimensions of the feature maps, helping to reduce computation and control overfitting. Max pooling is commonly used to retain the most important features.
Fully Connected Layers: After several convolutional and pooling layers, the feature maps are flattened and fed into fully connected layers to produce the final output (e.g., class probabilities).
How it works: CNNs automatically learn to extract hierarchical features from images, starting from low-level features (like edges) in the initial layers to more complex features (like objects) in deeper layers.
Use cases: Image classification, object detection (e.g., YOLO, Faster R-CNN), segmentation (e.g., U-Net), and more.
13. What is a recurrent neural network (RNN), and when is it used?
Answer: A Recurrent Neural Network (RNN) is a type of neural network designed for processing sequential data. Unlike traditional feedforward neural networks, RNNs have loops that allow information to persist, making them suitable for tasks where data is dependent on previous inputs.
How it works: RNNs use the output from the previous time step as input for the current time step, allowing the network to have "memory" of previous inputs.
Challenges: Vanilla RNNs often suffer from vanishing gradients, making it difficult to learn long-term dependencies.
Variants:
LSTM (Long Short-Term Memory): A specialized type of RNN designed to capture long-range dependencies by using gates (forget, input, and output gates) to control the flow of information.
GRU (Gated Recurrent Unit): A simplified version of LSTM, with fewer gates but similar performance.
Use cases: RNNs are used in time-series forecasting, natural language processing (NLP) tasks like machine translation, speech recognition, and sequence generation.
14. What are the different types of learning algorithms?
Answer: There are three main types of learning algorithms in machine learning:
Supervised Learning:
Description: The model is trained on labeled data, where both the input and the output are known. The goal is to learn a mapping from inputs to outputs.
Examples: Linear regression, decision trees, support vector machines (SVMs), and neural networks.
Use cases: Classification (e.g., spam detection), regression (e.g., predicting house prices).
Unsupervised Learning:
Description: The model is trained on unlabeled data. The goal is to find hidden patterns or structures within the data.
Examples: Clustering (e.g., K-means, hierarchical clustering), dimensionality reduction (e.g., PCA, t-SNE).
Use cases: Market segmentation, anomaly detection, data compression.
Reinforcement Learning:
Description: The model learns through interactions with an environment, receiving feedback in the form of rewards or penalties. The goal is to maximize cumulative rewards over time.
Examples: Q-learning, Deep Q-networks (DQN), Proximal Policy Optimization (PPO).
Use cases: Game playing (e.g., AlphaGo), robotic control, self-driving cars.
15. What is model interpretability, and why is it important?
Answer: Model interpretability refers to the ability to understand and explain how a machine learning model makes its predictions. Interpretability is particularly important in sensitive or regulated industries (like healthcare, finance, and legal domains), where stakeholders need to trust and understand the model’s decisions.
Importance:
Trust: Models that are interpretable build trust with users and decision-makers.
Debugging: Interpretability helps in understanding why a model may be making incorrect predictions and aids in debugging the model.
Compliance: In some sectors, regulations (like GDPR) require that model predictions be explainable, particularly when they affect individuals' lives (e.g., loan approvals, hiring decisions).
Interpretability techniques:
Feature importance: Measures how much each feature contributes to the final prediction.
LIME (Local Interpretable Model-agnostic Explanations): Explains individual predictions by approximating the model locally with a simpler, interpretable model.
SHAP (SHapley Additive exPlanations): Provides consistent and accurate feature importance values by distributing the prediction among the features based on Shapley values from game theory.
Trade-off: Often, more interpretable models (like linear regression) are simpler but may perform worse on complex tasks compared to more complex models (like deep neural networks), which are harder to interpret.
6. Behavioral and Cultural Fit Questions
In addition to technical expertise, top-tier companies place great importance on cultural fit and behavioral skills. These questions assess your soft skills, such as problem-solving, teamwork, leadership, and how you handle challenging situations. Often, companies use frameworks like the STAR method (Situation, Task, Action, Result) to evaluate your answers, and it’s important to structure your responses accordingly. Below are 10 common behavioral and cultural fit questions in ML interviews, along with tips on how to answer them.
1. Tell me about a time when you dealt with a challenging project.
What they’re looking for:
Your ability to handle adversity and navigate through challenges, both technical and interpersonal.
How to answer (STAR method):
Situation: Describe the challenging project. Was it an ML project with tight deadlines, difficult datasets, or complex algorithms?
Task: What was your role in the project? What was the specific problem that you needed to solve?
Action: Describe the steps you took to overcome the challenge. Did you break the project into smaller tasks, consult with peers, or apply creative problem-solving techniques?
Result: Explain the outcome. Did the project succeed? What did you learn from the experience?
2. Describe an instance where you had to advocate for an unpopular decision.
What they’re looking for:
Your leadership skills, ability to communicate effectively, and resilience in supporting decisions that may not initially have been well-received.
How to answer:
Situation: Describe the decision you had to advocate for. Perhaps it was choosing a different ML model or proposing a novel approach to a problem.
Task: Explain why the decision was unpopular. Did it involve significant risk or challenge existing methodologies?
Action: Detail how you presented your case. Did you use data to back your decision, or present a prototype to demonstrate effectiveness?
Result: Explain the final outcome. Did the team eventually agree? What was the impact of the decision?
3. Tell me about a time when you had to work under tight deadlines.
What they’re looking for:
Your time management skills, ability to work efficiently under pressure, and how well you manage stress.
How to answer:
Situation: Talk about a project where deadlines were critical, such as preparing an ML model for deployment or delivering insights from a dataset for a business decision.
Task: What was your specific responsibility? Was it coding, training a model, or analyzing data?
Action: Describe how you prioritized tasks, delegated responsibilities (if applicable), and maintained focus.
Result: Share the outcome. Did you meet the deadline? How did your performance impact the team or the project?
4. Give an example of a time when you worked in a cross-functional team.
What they’re looking for:
Your ability to collaborate with people from different backgrounds, such as product managers, data engineers, or business analysts, and how well you communicate complex ML concepts to non-technical stakeholders.
How to answer:
Situation: Describe the project and the different teams involved. Maybe you worked on integrating an ML model with a software application.
Task: What was your role in communicating ML concepts or ensuring the model aligned with business goals?
Action: Highlight how you bridged the gap between technical and non-technical teams. Did you hold meetings, create documentation, or present visualizations?
Result: Explain the impact. Was the collaboration successful, and how did it benefit the project?
5. Tell me about a time when you failed. How did you handle it?
What they’re looking for:
Your resilience and ability to learn from mistakes, as well as how you recover and prevent similar issues in the future.
How to answer:
Situation: Describe a project where something didn’t go as planned. Perhaps a model didn’t perform as expected, or a system you designed had scaling issues.
Task: What was your responsibility in the failure?
Action: Detail the steps you took after realizing the failure. Did you analyze the problem, seek feedback, or try a new approach?
Result: Focus on the lessons learned and how you applied them to future projects.
6. How do you handle disagreements in a team setting?
What they’re looking for:
Your interpersonal skills, ability to resolve conflict, and maintain a collaborative working environment.
How to answer:
Situation: Describe a time when you had a disagreement with a colleague or team member. Perhaps it was related to the direction of a project or the approach to solving an ML problem.
Task: Explain the nature of the disagreement.
Action: Outline how you handled the situation. Did you listen to the other person’s perspective, present your case with evidence, or suggest a compromise?
Result: Describe the outcome. Was the disagreement resolved, and what was the impact on the team or project?
7. Tell me about a time when you led a team or project.
What they’re looking for:
Your leadership skills, ability to motivate and guide a team, and how well you manage resources and deadlines.
How to answer:
Situation: Describe the project and your leadership role. Maybe you led the development of an ML model or managed an engineering team.
Task: What was your responsibility in leading the team? Did you set goals, manage timelines, or delegate tasks?
Action: Discuss how you organized the team, addressed challenges, and ensured progress.
Result: Share the outcome. Did the project succeed? How did your leadership contribute to the team’s success?
8. Give an example of how you handle stress in high-pressure situations.
What they’re looking for:
Your ability to manage stress without compromising the quality of your work, and how you stay focused during challenging times.
How to answer:
Situation: Describe a high-pressure scenario, such as working on a last-minute feature for an ML model deployment.
Task: What was the challenge, and how did the pressure impact the team or the project?
Action: Explain the strategies you used to handle stress—whether it was breaking tasks into manageable parts, staying organized, or taking breaks to clear your mind.
Result: Share how you successfully delivered the project and what you learned about managing stress.
9. Tell me about a time when you improved a process or workflow in your team.
What they’re looking for:
Your problem-solving skills and ability to find efficiencies that positively impact the team's productivity.
How to answer:
Situation: Describe the existing workflow that needed improvement. Maybe it was related to the ML model development pipeline or the way data was pre-processed.
Task: What was your role in identifying inefficiencies and suggesting improvements?
Action: Detail the steps you took to implement the improvement. Did you automate a task, reduce redundancies, or introduce new tools?
Result: Explain the positive impact on the team's productivity, accuracy, or morale.
10. How do you prioritize tasks when working on multiple projects?
What they’re looking for:
Your time management skills and how you balance competing priorities without sacrificing quality.
How to answer:
Situation: Describe a time when you had to manage multiple projects, such as building an ML model while supporting ongoing data analysis tasks.
Task: What were the competing priorities, and how did you manage the workload?
Action: Explain how you prioritized tasks—did you use tools like a task manager, delegate some responsibilities, or communicate with stakeholders to set realistic expectations?
Result: Share the outcome. How did prioritization help you complete tasks on time and to a high standard?
7. How InterviewNode Can Help
At InterviewNode, we specialize in helping software engineers and machine learning professionals prepare for rigorous interviews at top-tier companies like Google, Amazon, Meta, and Microsoft. Here’s how we can help you succeed:
Mock Interviews: Practice with real industry professionals who have experience working at top tech companies. Get valuable feedback on your coding, system design, and ML domain skills.
Curated ML-Specific Questions: Access a library of handpicked machine learning interview questions designed to challenge you across coding, system design, and domain-specific topics.
Personalized Feedback: After each mock interview or practice session, receive detailed feedback on your strengths and areas of improvement, along with actionable insights to refine your approach.
Resume Review: Optimize your resume to highlight the most relevant experiences and skills for machine learning roles, ensuring you stand out in the applicant pool.
Interview Simulation: Simulate the real interview environment with timed questions and problem-solving challenges to build confidence and improve performance under pressure.
With the right preparation and guidance from InterviewNode, you’ll be equipped to tackle the most challenging ML interviews and land your dream job at a top company.
8. Conclusion
Machine learning interviews at top-tier companies are challenging but entirely manageable with the right preparation. By reviewing and practicing the 50 most frequently asked questions in coding, system design, ML theory, and behavioral fit, you’ll build the necessary skills and confidence to stand out in the interview process. Remember that success in these interviews comes from a balance of technical expertise and effective communication.
To further improve your chances, sign up for mock interviews and personalized feedback sessions with InterviewNode—your partner in landing that coveted ML role.
Ready to take the next step? Join the free webinar and get started on your path to an ML engineer.
Comments