
Your Ultimate Guide to Common ML Interview Questions at Google and OpenAI
Jul 30
3 min read
0
22
0
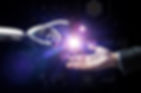
Preparing for machine learning (ML) engineering roles at top companies like Google and OpenAI requires a thorough understanding of core concepts, coding skills, and system design. Below are some of the most common ML interview questions you might encounter at these companies, along with strategies to solve them.
Optimization Algorithms:
Question: How does the Adam optimization algorithm differ from other optimization methods?
Strategy: Explain Adam’s unique features, such as combining the benefits of AdaGrad and RMSProp, adaptive learning rates, and efficiency in handling sparse gradients. Highlight its advantages for large datasets and complex architectures.
System Design:
Question: How would you design Google Home?
Strategy: Focus on diverse acoustic environments, natural language processing, and integration with the Google ecosystem. Discuss training deep-learning models with diverse voice data and iterating based on user feedback.
Ensemble Methods:
Question: What classifier should you use as the meta-classifier in a stacking model for a spam classifier?
Strategy: Choose logistic regression for its effectiveness in binary classification tasks. Explain how it refines predictions by interpreting base classifiers' outputs as features.
Probability and Coding:
Question: Write a function that returns a key at random with a probability proportional to the weights.
Strategy: Use Python’s random.choices() function to perform random selection based on the provided weights.
Clustering Algorithms:
Question: Prove that a k-means clustering algorithm will converge in a finite number of steps.
Strategy: Discuss the finite number of data points and cluster assignments, and how the algorithm iteratively minimizes the distance between points and centroids until convergence.
OpenAI ML Interview Questions
Natural Language Processing (NLP):
Question: Explain how BERT (Bidirectional Encoder Representations from Transformers) works.
Strategy: Describe the transformer architecture, bidirectional training, and applications in understanding the context of words in a sentence.
Reinforcement Learning:
Question: Describe the Q-learning algorithm.
Strategy: Explain the concept of Q-values, the update rule for Q-values using the Bellman equation, and the trade-off between exploration and exploitation.
Model Evaluation:
Question: Which cross-validation technique would you suggest for a time-series dataset and why?
Strategy: Recommend time series cross-validation, which respects temporal dependencies by only using past data to predict future values.
Computer Vision:
Question: What is the state-of-the-art object detection algorithm YOLO?
Strategy: Discuss YOLO’s single pass through a CNN for real-time object detection, predicting class probabilities, and boundary boxes simultaneously.
Strategies for Solving Common ML Interview Problems
Understand the Problem:
Carefully read the problem statement and identify key components.
Ask clarifying questions if the problem is not fully understood.
Break Down the Solution:
Divide the problem into smaller, manageable parts.
Write pseudocode or outline steps before coding.
Optimize Your Code:
Focus on writing efficient, clean, and bug-free code.
Consider time and space complexity when designing your solution.
Practice Common Algorithms:
Regularly practice problems involving graphs, trees, arrays, and dynamic programming.
Use platforms like LeetCode, HackerRank, and Interview Query for practice.
Review Machine Learning Concepts:
Refresh your understanding of core ML concepts like optimization algorithms, ensemble methods, and neural networks.
Study system design principles, especially for large-scale ML systems.
Example Code
Here’s an example of solving a weighted random selection problem in Python:
import random
def weighted_random_choice(weighted_dict):
keys = list(weighted_dict.keys())
weights = list(weighted_dict.values())
return random.choices(keys, weights=weights, k=1)[0]
# Example usage
weighted_dict = {'a': 1, 'b': 2, 'c': 3}
print(weighted_random_choice(weighted_dict))
This function selects a key from weighted_dict based on the provided weights using the random.choices() method.
By focusing on these strategies and practicing regularly, you can enhance your chances of success in ML engineering interviews at Google, OpenAI, and other top tech companies.